GUI Scripting Guide
Overview
GUI stands for Graphical User Interface. Unity's GUI system is called UnityGUI. UnityGUI allows you to create a huge variety of GUIs complete with functionality very quickly and easily. Rather than creating a GUI object, manually positioning it, and then writing a script that handles its functionality, you do all of this at once in a small amount of code. This works by creating GUI Controls, which are instantiated, positioned, and defined all at once.
For example, the following code will create a fully functional button from scratch:
function OnGUI () { if (GUI.Button (Rect (10,10,150,100), "I am a button")) { print ("You clicked the button!"); } }
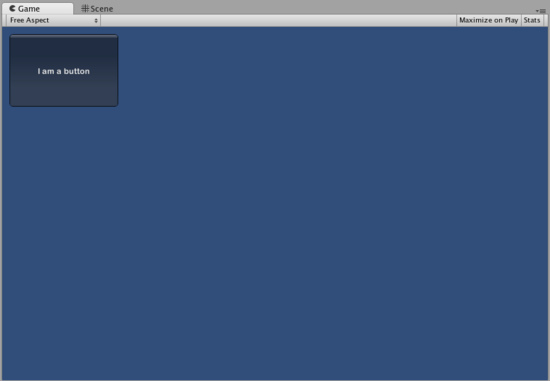
This is the button created by the code above
Although this example is very simple, there are very powerful and complex techniques available for use in UnityGUI. It is a broad subject, and the following sections will help you get up to speed as quickly as possible. This guide can be read straight through, or used as reference material.
UnityGUI Basics
This section covers the important concepts of UnityGUI, giving you an overview as well as a bunch of working examples you can paste into your own projects. UnityGUI is very friendly to play with, so this is a good place to get started.
Controls
This section lists every available Control in UnityGUI. It is complete with code examples and images.
Customization
Creating functional GUI controls would not be as useful if their appearances couldn't be customized. Thankfully, we've thought of this. All controls in UnityGUI can be customized with GUIStyles and GUISkins. This section explains how to use them.
Layout Modes
UnityGUI features two ways to arrange your GUIs. You can manually place each control on the screen, or you can use an Automatic Layout system which works a lot like HTML tables. Using one system or the other is as simple as using a different class, and you can mix the two together. This section explains the functional differences between the two systems, including examples.
Extending UnityGUI
UnityGUI is very easy to extend with new Control types. This chapter shows you how to make simple compound controls - complete with integration into Unity's event system.
Extending Unity Editor
The main Unity editor is written using UnityGUI. It is completely extensible using the same code as you would use for in-game GUI. In addition, there are a bunch of Editor specific GUI widgets to help you out when creating custom editor GUI.
Page last updated: 2010-08-19